Contents
- Development Platform
- Introduction
- Prepare the stage
- Creating the projects and necessary files
- Configure the WCF for XBAP
- Consume WCF Service from XBAP
- Debugging WCF Service
- Deploying your solution
- Using the WsHttpBinding configuration
- A quick discussion on Data Transfer Limit
- Conclusion
Development Platform
- Visual Studio 2008
- Dot net framework 3.5
- IIS 5.1~7
- IE7/Firefox 2.x
- Asp.net 2.0
- Windows vista/xp
Introduction
Windows presentation foundation in short, wpf is Microsoft’s new user interface technology. Wpf introduced with the release of dot net framework 3.0. Wpf comes with lot of promises, for example, it can be used to show very rich graphics centric 2D and 3D models. WPF uses the client machines graphics capability to full extent. For more information about wpf please visit www.windowclient.net. In this article we will discuss about using wpf in web! It sounds a very strange wish to accomplish. But it’s true; wpf can be used in browser. This special browser centric package called WPF Browser Application. XBAP stands for XAML Browser Application which is the eventual output of WPF browser application. The main purpose of XBAP is to introduce a fat client application which runs on client side and provide the user an ease of desktop application in web. XBAP use the resources of client machine, that’s why .net framework must be installed in client machine to run WPF browser application. Even though XBAP uses client machine's resource and memory but it run in a sandbox environment, this mode of sandbox called the partial trust mode. XBAP in dot net 3.5 have few improvement over 3.0. Using WCF in partial trust mode is one of them. In this article we will see the implemention of WCF service in XBAP partial trust mode.
Prepare the stage
Learning by example is always a very good way of learning new things, that’s why we are going to develop a simple XAML browser application in which we will display a to-do list and notes of a user and of course going to use WCF to communicate with our data layer. Few things must be clear before jumped in to the stage. In partial trust mode XBAP can consume WCF service from the site of origin only. So we cannot use a cross domain WCF service from XBAP. Another restriction we have in case of WCF binding configuration, we can use only BasicHttpBinding and WSHttpBinding in partial trust mode. For retrieving, creating, deleting and updating tasks we will use BasicHttpBinding. On the other hand to retrieving, creating, deleting and updating notes we will use WsHttpBinding. Let’s go through the projects templates first. We will of course create a WPF Browser application Project, Next we are going to create a WCF Service Application Project and Finally we are going to develop a simple web site (web application project of vs2008) where our browser application will be hosted. We will use a SQL express edition database in data service layer that is a “tododata.mdf” we will construct our own user base and user validation. WPF browser application will forward all request to a WCF web service. WCF service will do business logic validation and retrieve data from data access layer. Bellow here is a simple diagram to presented how the application architecture will be.
Creating the projects and necessary files
By now we already know that we are going to create three different projects. So let’s get started, we will start with creating a blank solution for our convenience. Go ahead and create a blank solution in vs2008 and save the solution to your convenient place with a convenient name. Now add a new WPF Browser application project to the solution. Then add a new WCF Service Application to the solution and lastly add a new web application project to the solution, that concludes the projects creation process. To save the data we will use sql express database, so let's go ahead and add a Sql server database file and name it to your convenient. Then create necessary tables for task, notes and users. Next we need a simple mechanism to retrieve, save and to modify data from the database, for this we are going to use LINQ. Add a dbml file to the wcf service project drag and drop the data tables from database explorer to the dbml. Next we have to define our service interfaces. We will have two services in the same wcf project one for notes (INoteService) and other one for tasks (ITaskService).
Configure the WCF for XBAP
If you are new to wcf I would like to suggest you to spend some time on msdn library learning wcf. So now we have our wcf service application “WcfService”. Now we are going to configure our wcf service so that our XBAP can consume this service. By default when users add a wcf service, visual studio automatically configure two end points for the service. We are going to modify the binding configuration for our service so that XBAP can communicate with it. Visual studio 2008 provides a nice tool to edit wcf service configurations, and the tool is “WCF Service Configuration Editor” we will edit service configurations with this tool, you can also modify the web.config manually to setup appropriate markup under service model section. By default when you right click on web.config of service no edit configuration tool is shown, to make it show first we have to run it from the tools menu of visual studio 2008. Click on the tools menu of the visual studio 2008 and click on wcf configuration menu. Now you can close the tool. Once you have opened the tool it will be available for the current session. Now right click on the web.config file of the wcf service project and select “Edit WCF configuration”. Configuration editor will pop up with the entire configuration that is defined in the web.config. In configuration editor on the left side you will find a tree with service information’s populated. Expand the services node and you will see a node named “Endpoints”. By default under endpoints node there are two pre-added endpoints, one endpoint configured with WsHttpBinding and another is configured with MaxHttpBinding. Now select the endpoint that is configured with WsHttpBinding. After selecting the endpoint, properties of that end point will be shown in property editor on the right side. Change the binding property to WsHttpBinding to BasicHttpBinding. Click on file and save and close the tool.
Now it’s time to host the service to a location so that our application can consume it from a fixed location. When we added the wcf service application by default visual studio 2005/2008 configure the service to run on visual studio development server. We are going to modify this to run from IIS. For this right click on wcf service project and select properties. After project properties window popup, navigate to web tab and change the server configuration under servers section from “Use Visual Studio Development server” to “Use IIS Web server” after that you need to click on create virtual directory button to finish the process. Now save the project. And you are all done configuring the service for you xbap. If you are in windows vista you can encounter with few errors, to overcome the problems you must run visual studio with “run as administrator” mode.
Consume WCF Service from XBAP
The hard part is now over, just add a service reference like normal procedure i.e. right click on project select “add service reference “ after add service reference window popup, click on discover button. You will see the service will be found and URL will be added to the address textbox. Click okay and you are all done.
Because of the previous action that is performed appropriate mark up will be added in the app.config under service model configurations section. The markup that is added look like this
<system.serviceModel>
<bindings>
<basicHttpBinding>
<binding name="BasicHttpBinding_IService1" closeTimeout="00:01:00"
openTimeout="00:01:00" receiveTimeout="00:10:00" sendTimeout="00:01:00"
allowCookies="false" bypassProxyOnLocal="false" hostNameComparisonMode="StrongWildcard"
maxBufferSize="65536" maxBufferPoolSize="524288" maxReceivedMessageSize="65536"
messageEncoding="Text" textEncoding="utf-8" transferMode="Buffered"
useDefaultWebProxy="true">
<readerQuotas maxDepth="32" maxStringContentLength="8192" maxArrayLength="16384"
maxBytesPerRead="4096" maxNameTableCharCount="16384" />
<security mode="None">
<transport clientCredentialType="None" proxyCredentialType="None"
realm="" />
<message clientCredentialType="UserName" algorithmSuite="Default" />
</security>
</binding>
</basicHttpBinding>
</bindings>
<client>
<endpoint address="http://munna.kaz.com.bd/TODO.SERVICE/Service1.svc"
binding="basicHttpBinding" bindingConfiguration="BasicHttpBinding_IService1"
contract="TodoServiceReference1.IService1" name="BasicHttpBinding_IService1" />
</client>
</system.serviceModel>
Debugging WCF Service
While developing XBAP sometimes developers need to debug the
application. Developer may needs to investigate what data is received
to the service and what data is transmitted from the service. Well,
since WCF works in XBAP only in partial trust mode it’s become a little
bit difficult, but not yet that much difficult. While debug from visual
studio 2008 we can set the “trust mode” to full trust. You can set
trust mode to full trust from security tab of project properties. This
will enable your WPF Project to debug in application in development
environment. But while deployment you must change trust mode back to
partial trust otherwise you will encounter with trust not granted error.
Deploying your solution
First of all developer needs to set XBAP’s security mode to partial
trust. In publish wizard first step is to specify where you want to
publish the binary. Browse and select a folder as convenience. Click
next will guide the user to select next option which is selection of
how the user will install the application. In this screen developer
needs to select “from a web site” option and specify the location of
the web site. Browse or put down the location of the web site. While
deploying your solution you must be careful about the deployment URL
and the URL or the WCF service that is defined in the app.confiq of the
XBAP. In app.config let’s say you have an end point with
http://localhost/wcfservice you should also put your XBAP deployment
URL as http://localhost/xbaphost. If you in app.config you have
localhost but you browse as http://{pcname}/xbaphost things won’t work
and you will end up with a code access security violation exception. So
you must remain consistent about the URL of WCF Service, XBAP Host, and
the URLS.
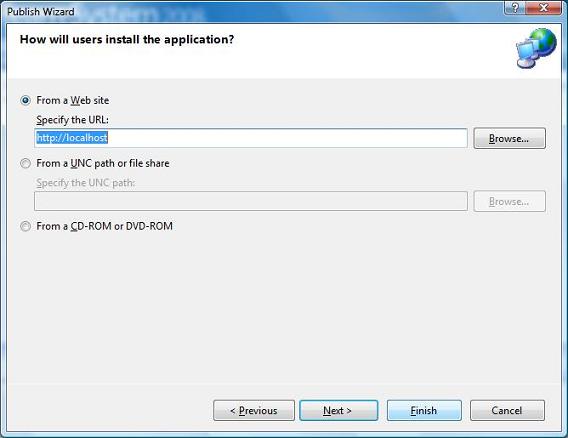
Using the WsHttpBinding Configuration
You can also use WsHttpBinding to communicate with the wcf also, but
few things need to configure to ensure that you don’t suffer from code
access security exception. If you want to use WsHttpBinding you don’t
have to create any new binding. Visual studio 2008 by default creates
two end points for a wcf service application one is WsHttpBinding and
another is MaxHttpBinding. What developer needs to do is to configure a
new binding configuration. To accomplish this edit the web.config of
WCF Service application. In default window you will see first endpoint
is configured with WsHttpBinding and under the endpoint a default
binding is configured, it should say “(Default) Click to Create” just
click to create new binding configuration and configure it not to use
reliable session and security mode to None. Bellow the service mode
configuration is provided for better understanding; eventually WCF
Configuration Editor will generate this sort of markup.
<system.serviceModel>
<bindings>
<wsHttpBinding>
<binding name="NewBinding0" messageEncoding="Text">
<reliableSession enabled="false"/>
<security mode="None">
</security>
</binding>
</wsHttpBinding>
</bindings>
<services>
<service behaviorConfiguration="TESTWCF.Service1Behavior" name="TESTWCF.Service1">
<endpoint address="" binding="wsHttpBinding" bindingConfiguration="NewBinding0"
contract="TESTWCF.IService1">
</endpoint>
<endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange" />
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="TESTWCF.Service1Behavior">
<!-- To avoid disclosing metadata information, set the value below to false and remove
the metadata endpoint above before deployment -->
<serviceMetadata httpGetEnabled="true"/>
<!-- To receive exception details in faults for debugging purposes, set the value below
to true. Set to false before deployment to avoid disclosing exception information -->
<serviceDebug includeExceptionDetailInFaults="false"/>
</behavior>
</serviceBehaviors>
</behaviors>
</system.serviceModel>
Make sure that you set enable reliable session to “false” and set
security mode to “none” in both client endpoint and service’s endpoint
binding configuration. Otherwise XBAP can suffer from code access
security exception.
A quick discussion on Data Transfer Limit
XBAP to WCF communication data transfer have few limitations in data
transfer size. If you are transferring huge list of objects that
exceeds the size specified in max buffer size and max receive size in
web.config application will cause receive limit exceed exception. You
can increase the size of the maxBufferSize and maxReceivedMessageSize
from web.config in binding configuration section of service, but
increasing its size will cause the application suffer from code access
permission exception. So a very straight forward way to escape from
this issue is to make your service in such a way so that you don’t
return data that exceed the size limit. And if you have huge data to
transfer, change the service contract in such a way, so that you can
transfer data in multiple requests, and in every request size limit
should remain under the maxBufferSize.
Conclusion
In few cases you will see that service is taking a little bit time
to respond to your request, and that can cause your application to
become irresponsive for few seconds. To escape from this problem you
can use background process to call the WCF service, and display a wait
screen to the user and hide the wait screen after the process finished
execution. That concludes a beginner introduction to xbap to wcf
communication.